QR and barcode scanner in React
- Introduction
- Install the library using npm
- Create a React component
- Use this plugin in your React App
- Passing around the callback
- Full code reference
- Credits
- Closing note
mebjas/html5-qrcode is a fairly used open source library for implementing QR Code or barcode scanner in a web application. There are several developers who have been using it under web-view for android projects as well. In this article I’ll be explaining how to use html5-qrcode with React so it’s easier for developers using popular React framework to these functionalities with ease.
Introduction
I don’t think ReactJs needs any explanation here and throughout this article I’ll assume the readers have familiarity with React
, Components
, state
, props
etc.
If you are just interested in implementing QR code or barcode scanning on web without react, I recommend you read this article
QR and barcode scanner using HTML and JavaScript
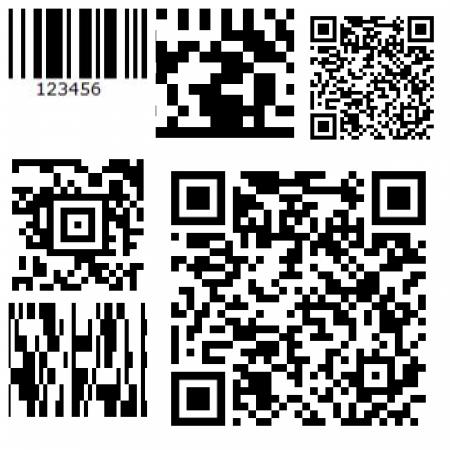
[ Read more ]
Install the library using npm
First, install the latest version of the library in your React project, using npm
npm i html5-qrcode
Create a React component
Next step is to create a React that abstracts most of the scanner implementation.
Let say we create a new file called Html5QrcodePlugin.jsx
// Html5QrcodePlugin.jsx
import { Html5QrcodeScanner } from "html5-qrcode";
import React from 'react';
const qrcodeRegionId = "html5qr-code-full-region";
class Html5QrcodePlugin extends React.Component {
render() {
return <div id={qrcodeRegionId} />;
}
componentWillUnmount() {
// TODO(mebjas): See if there is a better way to handle
// promise in `componentWillUnmount`.
this.html5QrcodeScanner.clear().catch(error => {
console.error("Failed to clear html5QrcodeScanner. ", error);
});
}
componentDidMount() {
// Creates the configuration object for Html5QrcodeScanner.
function createConfig(props) {
var config = {};
if (props.fps) {
config.fps = props.fps;
}
if (props.qrbox) {
config.qrbox = props.qrbox;
}
if (props.aspectRatio) {
config.aspectRatio = props.aspectRatio;
}
if (props.disableFlip !== undefined) {
config.disableFlip = props.disableFlip;
}
return config;
}
var config = createConfig(this.props);
var verbose = this.props.verbose === true;
// Suceess callback is required.
if (!(this.props.qrCodeSuccessCallback )) {
throw "qrCodeSuccessCallback is required callback.";
}
this.html5QrcodeScanner = new Html5QrcodeScanner(
qrcodeRegionId, config, verbose);
this.html5QrcodeScanner.render(
this.props.qrCodeSuccessCallback,
this.props.qrCodeErrorCallback);
}
};
export default Html5QrcodePlugin;
Important note: html5-qrcode is an actively developed library. It’s advisable to subscribe to updates at mebjas/html5-qrcode so you can keep the React library up to date.
Use this plugin in your React App
I’ll assume you have an App.js
that is the source component. You can add the new Component
we just created.
class App extends React.Component {
constructor(props) {
super(props);
// This binding is necessary to make `this` work in the callback.
this.onNewScanResult = this.onNewScanResult.bind(this);
}
render() {
return (<div>
<h1>Html5Qrcode React example!</h1>
<Html5QrcodePlugin
fps={10}
qrbox={250}
disableFlip={false}
qrCodeSuccessCallback={this.onNewScanResult}/>
</div>);
}
onNewScanResult(decodedText, decodedResult) {
// Handle the result here.
}
);
Passing around the callback
You might have seen the callback defined in the above section
// ... rest of the code
onNewScanResult(decodedText, decodedResult) {
// Handle the result here.
}
// ... rest of the code
Use this callback to define rest of your business logic. Let say your use-case is to pass the newly scanned result and print in on a <table>
, you’d want to pass around the data to a different component. You can find an example of this at scanapp-org/html5-qrcode-react.
Full code reference
The full example has been created at scanapp-org/html5-qrcode-react - you can use that as a good reference.
Credits
scanapp.org is a free online QR code and barcode reader for web built using this library - try it out.
Closing note
I have to admit, I am not React savvy, if you see errors or know of better way to do things please suggest it using the comments or send a pull request to scanapp-org/html5-qrcode-react.
Want to read more such similar contents?
I like to write articles on topic less covered on internet. They revolve around writing fast algorithms, image processing as well as general software engineering.
I publish many of them on Medium.
If you are already on medium - Please join 4200+ other members and Subscribe to my articles to get updates as I publish.
If you are not on Medium - Medium has millions of amazing articles from 100K+ authors. To get access to those, please join using my referral link. This will give you access to all the benefits of Medium and Medium shall pay me a piece to support my writing!
Thanks!