HTML5 QR Code scanner
ScanApp - Free QR code and barcode scanner for web
scanapp.org is a free online QR code and barcode reader for web built using this library - try it out.
scanapp.org is a free online QR code and barcode reader for web built using this library - try it out.
The latest article: QR and barcode scanner using HTML and JavaScript
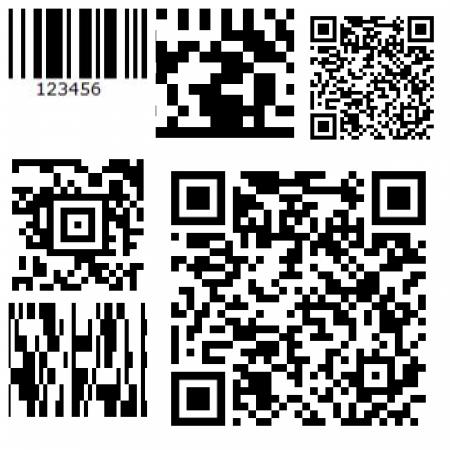
version 2.x.x
. I'll also list out the new APIs and capabilities that developers can use to integrate a more powerful code scanning capability to their web pages or apps
[ Read more ]
How to use?
You can either use
Html5QrcodeScanner
which takes of end to end
user interface and functionality, or you can use Html5Qrcode
with your own user interface. This demo is build using Html5QrcodeScanner
.
[1] Add javascript to your webpage either using npm

Or include the JavaScript script directly using
<script src="html5-qrcode.min.js"></script>
.
[2] Add an empty div where you want to place the qrcode scanner
<div style="width: 500px" id="reader"></div>
[3] Initialize
function onScanSuccess(decodedText, decodedResult) {
// Handle on success condition with the decoded text or result.
console.log(`Scan result: ${decodedText}`, decodedResult);
}
var html5QrcodeScanner = new Html5QrcodeScanner(
"reader", { fps: 10, qrbox: 250 });
html5QrcodeScanner.render(onScanSuccess);
[4] Optional - Stop scanning after the code is scanned?
You can useHtml5QrcodeScanner#clear()
method to stop scanning after the code is scanned. Here's an example:
var html5QrcodeScanner = new Html5QrcodeScanner(
"reader", { fps: 10, qrbox: 250 });
function onScanSuccess(decodedText, decodedResult) {
// Handle on success condition with the decoded text or result.
console.log(`Scan result: ${decodedText}`, decodedResult);
// ...
html5QrcodeScanner.clear();
// ^ this will stop the scanner (video feed) and clear the scan area.
}
html5QrcodeScanner.render(onScanSuccess);
[5] Optional - Handle scan failure?
You can handle scan failure or video failure using failure callback. Here's an example:function onScanSuccess(decodedText, decodedResult) {
// Handle on success condition with the decoded text or result.
console.log(`Scan result: ${decodedText}`, decodedResult);
}
function onScanError(errorMessage) {
// handle on error condition, with error message
}
var html5QrcodeScanner = new Html5QrcodeScanner(
"reader", { fps: 10, qrbox: 250 });
html5QrcodeScanner.render(onScanSuccess, onScanError);
[6] More configurations
The library supports certain configurations that allows you to change the behavior. Visit the Github documentation to learn more about different configurations.Configuration | Description |
---|---|
fps |
Integer, Example = 10 A.K.A frame per second, the default value for this is 2 but it can be increased to get faster scanning. Increasing too high value could affect performance. Value >1000 will simply fail.
|
qrbox |
Integer, Example = 250 Use this property to limit the region of the viewfinder you want to use for scanning. The rest of the viewfinder would be shaded. For example by passing config { qrbox : 250 } .
|
aspectRatio |
Float, Example 1.777778 for 16:9 aspect ratio Use this property to render the video feed in a certain aspect ratio. Passing a nonstandard aspect ratio like 100000:1 could lead to the video feed not even showing up. If you do not pass any value, the whole viewfinder would be used for scanning. Note: this value has to be smaller than the width and height of the QR code HTML element. |
disableFlip |
Boolean (Optional), default = false By default, the scanner can scan for horizontally flipped QR Codes. This also enables scanning QR code using the front camera on mobile devices which are sometimes mirrored. This is false by default and I recommend changing this only if: |
formatsToSupport |
Html5QrcodeSupportedFormats (Optional) By default, both camera stream and image files are scanned against all the supported code formats. Both Html5QrcodeScanner and Html5Qrcode classes can be
configured to only support a subset of supported formats. Supported formats are defined in enum Html5QrcodeSupportedFormats.Read more... |
FAQs
Is this supported on smartphones?Yes! the solution is based on vanilla HTML5 and javascript. This should work on any HTML5 supported browser.
Full example on how to use this?
Check source code of this page or checkout this gist where I have copied js code.
I copied the demo code it's not working for me?
Few identified reasons so far:
Where can I read more about this, is there a reference article?
- Camera access only work for
https
and nothttp
except forlocalhost or local server
. - You cannot test this by opening the HTML file, it should be backed by a server — How to allow Chrome to access my camera on localhost?.
Apart from the documentation on the github project, following blog article may be helpful.
The little QR code scanning library I have been maintaining since 2015 has been getting more attention recently. And with power came responsibilities, bugs, and feature requests. Some of the key features requested by developers were more reliable scanning and the ability to scan different types of bar codes. With
This article lists out everything new in
html5-qrcode helps developer to integrate QR code scanning in HTML5 based applications. It abstracts camera access and usage on different browsers and simplify QR Code scanning for developers. The primary goal of the library is cross-platform support across different OS versions and Browsers. One of the key problems with cross-platform support was some browsers in Android and many browsers in iOS (almost all other than Safari which are based on WebKit for iOS) did not support camera access. This prevents users from doing inline QR Code scanning which is the primary feature of the library. To mitigate this I have added support for scanning local media on the device, and it implicitly adds support for capturing QR Code using default camera on the device and scanning it. This is an upgrade to the existing library — Read this article to learn more. In this article I have explained now file-based scanning works and how to use it.
[ Read more ]
QR and barcode scanner using HTML and Javascript
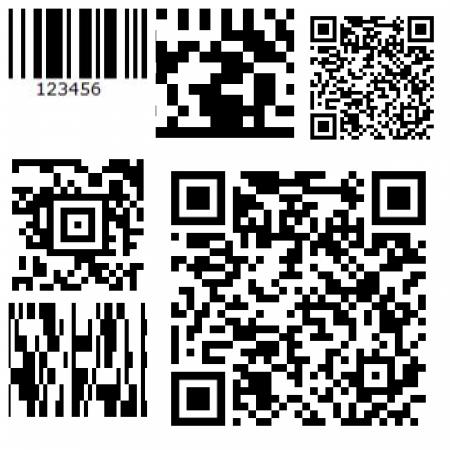
version 2.0.0
onwards developers can scan different types of 1D codes (bar codes) and 2D codes (like QR codes or AZTEC).This article lists out everything new in
version 2.x.x
. I'll also list out the new APIs and capabilities that developers can use to integrate a more powerful code scanning capability to their web pages or apps
[ Read& more ]
HTML5 QR Code scanning with javascript - Support for scanning the local file and using default camera added (v1.0.5)
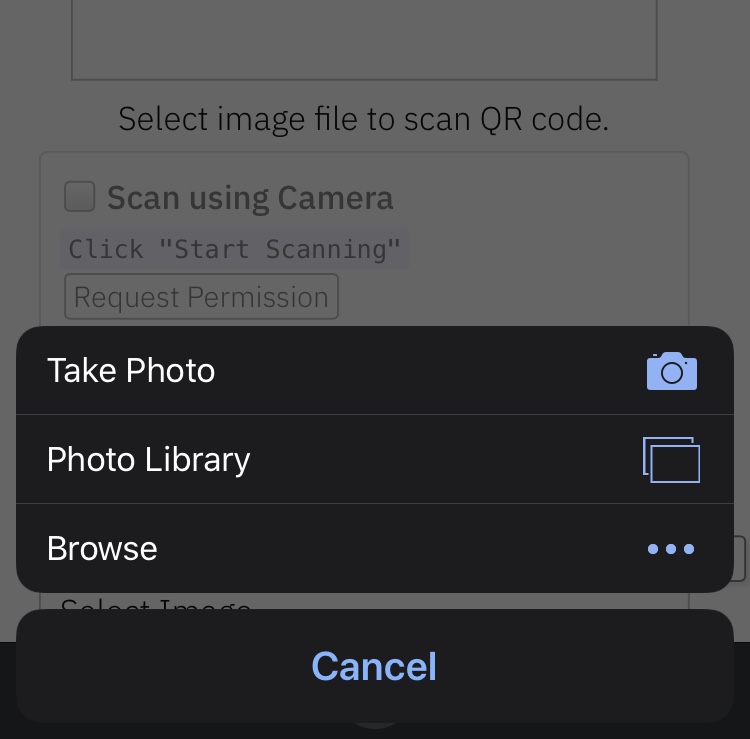
References
- Project on Github - mebjas/html5-qrcode
- How to use - blog.minhazav.dev/QR-and-barcode-scanner-using-html-and-javascript/
- File based scanning - blog.minhazav.dev/HTML5-QR-Code-scanning-support-for-local-file-and-default-camera
- Edit images before uploading - MediaPanda - edit images, securely.